11.03.2019, 14:15
So I made a custom damage system based off of this guide https://sampforum.blast.hk/showthread.php?tid=558839, but there is an issue.
If I pistol whip someone (punch someone with a gun) it bases it off torso damage. Basically, I can punch someone and hit 40 now. How do I solve this issue?
Example:
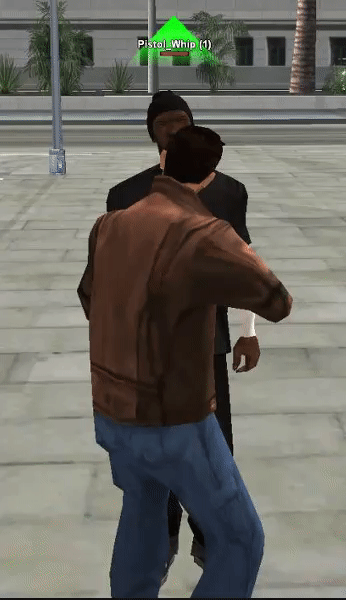
See code below.
If I pistol whip someone (punch someone with a gun) it bases it off torso damage. Basically, I can punch someone and hit 40 now. How do I solve this issue?
Example:
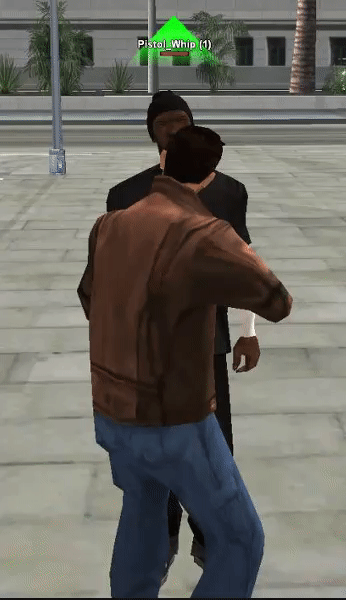
See code below.
PHP код:
#define BODY_PART_TORSO 3
#define BODY_PART_GROIN 4
#define BODY_PART_LEFT_ARM 5
#define BODY_PART_RIGHT_ARM 6
#define BODY_PART_LEFT_LEG 7
#define BODY_PART_RIGHT_LEG 8
#define BODY_PART_HEAD 9
my_SetPlayerArmour(playerid, Float:amount)
{
if(amount < 0.0)
{
amount = 0.0;
}
return SetPlayerArmour(playerid, amount);
}
#if defined _ALS_SetPlayerArmour
#undef SetPlayerArmour
#else
#define _ALS_SetPlayerArmour
#endif
#define SetPlayerArmour my_SetPlayerArmour
public OnPlayerConnect(playerid)
{
SetPlayerTeam(playerid, 0);
return 1;
}
public OnPlayerTakeDamage(playerid, issuerid, Float:amount, weaponid, bodypart)
{
new Float: health,Float: armour;
GetPlayerHealth(playerid, health);
GetPlayerArmour(playerid, armour);
switch(weaponid) // Starts the switch, and switches through the variable "weaponid". (declared in callback)
{
case 24: // Desert Eagle weapon ID
{
switch(armour)
{
case 0:
{
switch(bodypart)
{
case 3: SetPlayerHealth(playerid, health - 40); // Torso.
case 4: SetPlayerHealth(playerid, health - 18); // Groin.
case 5: SetPlayerHealth(playerid, health - 8); // Left Arm.
case 6: SetPlayerHealth(playerid, health - 8); // Right Arm.
case 7: SetPlayerHealth(playerid, health - 8); // Left Leg.
case 8: SetPlayerHealth(playerid, health - 8); // Right Leg.
case 9: SetPlayerHealth(playerid, health - 50); // Head.
}
}
default:
{
switch(bodypart)
{
case 3: SetPlayerArmour(playerid, armour - 30); // Torso.
case 4: SetPlayerArmour(playerid, armour - 14); // Groin.
case 5: SetPlayerArmour(playerid, armour - 6); // Left Arm.
case 6: SetPlayerArmour(playerid, armour - 6); // Right Arm.
case 7: SetPlayerArmour(playerid, armour - 6); // Left Leg.
case 8: SetPlayerArmour(playerid, armour - 6); // Right Leg.
case 9: SetPlayerArmour(playerid, armour - 40); // Head.
}
}
}
}
}
}
return 1;
}