30.09.2017, 16:34
Gradient Textdraw?
30.09.2017, 17:03
Those are lots of small textdraws next to each other (best use Sprites for precise squares).
The gradient can easily be calculated by taking Color 1 RGB values and Color 2 RBG values (ignore transparency) and then fade them from COLOR_1 to COLOR_2.
If you change the TextDraw to be a Sprite (LD_BUM:blkdot is nice for this) it should work.
The gradient can easily be calculated by taking Color 1 RGB values and Color 2 RBG values (ignore transparency) and then fade them from COLOR_1 to COLOR_2.
Код:
#define FADE_COLOR_1 0xFFFF00FF // Colors to fade #define FADE_COLOR_2 0xFF00FFFF #define MAX_FADE_DRAWS 10 // Number of TextDraws // When creating: new r1, g1, b1, r2, g2, b2; r1 = (FADE_COLOR_1 >> 24) & 255; // Get R,G,B from COLOR_1 g1 = (FADE_COLOR_1 >> 16) & 255; b1 = (FADE_COLOR_1 >> 8) & 255; r2 = (FADE_COLOR_2 >> 24) & 255; // Get R,G,B from COLOR_2 g2 = (FADE_COLOR_2 >> 16) & 255; b2 = (FADE_COLOR_2 >> 8) & 255; new delta_r = (r2 - r1) / MAX_FADE_DRAWS, // Delta R,G,B (for every step, this is the color that we add to COLOR_1) delta_g = (g2 - g1) / MAX_FADE_DRAWS, delta_b = (b2 - b1) / MAX_FADE_DRAWS; for(new i = 0; i < MAX_FADE_DRAWS; i ++) { new rgba = 0x000000FF + ((r1 + delta_r*i) * 256 * 256 * 256) + ((g1 + delta_g*i) * 256 * 256) + ((b1 + delta_b*i) * 256); // Calculate HEX Color (including Alpha!) new Text:FadeText = TextDrawCreate(640.0 / MAX_FADE_DRAWS * i, 400.0, "..."); // Make this a TextDraw Sprite and save the IDs in an Array to be able to show them later TextDrawColor(FadeText, rgba); }
30.09.2017, 17:41
Quote:
Those are lots of small textdraws next to each other (best use Sprites for precise squares).
The gradient can easily be calculated by taking Color 1 RGB values and Color 2 RBG values (ignore transparency) and then fade them from COLOR_1 to COLOR_2. Код:
#define FADE_COLOR_1 0xFFFF00FF // Colors to fade #define FADE_COLOR_2 0xFF00FFFF #define MAX_FADE_DRAWS 10 // Number of TextDraws // When creating: new r1, g1, b1, r2, g2, b2; r1 = (FADE_COLOR_1 >> 24) & 255; // Get R,G,B from COLOR_1 g1 = (FADE_COLOR_1 >> 16) & 255; b1 = (FADE_COLOR_1 >> 8) & 255; r2 = (FADE_COLOR_2 >> 24) & 255; // Get R,G,B from COLOR_2 g2 = (FADE_COLOR_2 >> 16) & 255; b2 = (FADE_COLOR_2 >> 8) & 255; new delta_r = (r2 - r1) / MAX_FADE_DRAWS, // Delta R,G,B (for every step, this is the color that we add to COLOR_1) delta_g = (g2 - g1) / MAX_FADE_DRAWS, delta_b = (b2 - b1) / MAX_FADE_DRAWS; for(new i = 0; i < MAX_FADE_DRAWS; i ++) { new rgba = 0x000000FF + ((r1 + delta_r*i) * 256 * 256 * 256) + ((g1 + delta_g*i) * 256 * 256) + ((b1 + delta_b*i) * 256); // Calculate HEX Color (including Alpha!) new Text:FadeText = TextDrawCreate(640.0 / MAX_FADE_DRAWS * i, 400.0, "..."); // Make this a TextDraw Sprite and save the IDs in an Array to be able to show them later TextDrawColor(FadeText, rgba); } |
30.09.2017, 18:30
Quote:
Those are lots of small textdraws next to each other (best use Sprites for precise squares).
The gradient can easily be calculated by taking Color 1 RGB values and Color 2 RBG values (ignore transparency) and then fade them from COLOR_1 to COLOR_2. Код:
#define FADE_COLOR_1 0xFFFF00FF // Colors to fade #define FADE_COLOR_2 0xFF00FFFF #define MAX_FADE_DRAWS 10 // Number of TextDraws // When creating: new r1, g1, b1, r2, g2, b2; r1 = (FADE_COLOR_1 >> 24) & 255; // Get R,G,B from COLOR_1 g1 = (FADE_COLOR_1 >> 16) & 255; b1 = (FADE_COLOR_1 >> 8) & 255; r2 = (FADE_COLOR_2 >> 24) & 255; // Get R,G,B from COLOR_2 g2 = (FADE_COLOR_2 >> 16) & 255; b2 = (FADE_COLOR_2 >> 8) & 255; new delta_r = (r2 - r1) / MAX_FADE_DRAWS, // Delta R,G,B (for every step, this is the color that we add to COLOR_1) delta_g = (g2 - g1) / MAX_FADE_DRAWS, delta_b = (b2 - b1) / MAX_FADE_DRAWS; for(new i = 0; i < MAX_FADE_DRAWS; i ++) { new rgba = 0x000000FF + ((r1 + delta_r*i) * 256 * 256 * 256) + ((g1 + delta_g*i) * 256 * 256) + ((b1 + delta_b*i) * 256); // Calculate HEX Color (including Alpha!) new Text:FadeText = TextDrawCreate(640.0 / MAX_FADE_DRAWS * i, 400.0, "..."); // Make this a TextDraw Sprite and save the IDs in an Array to be able to show them later TextDrawColor(FadeText, rgba); } |
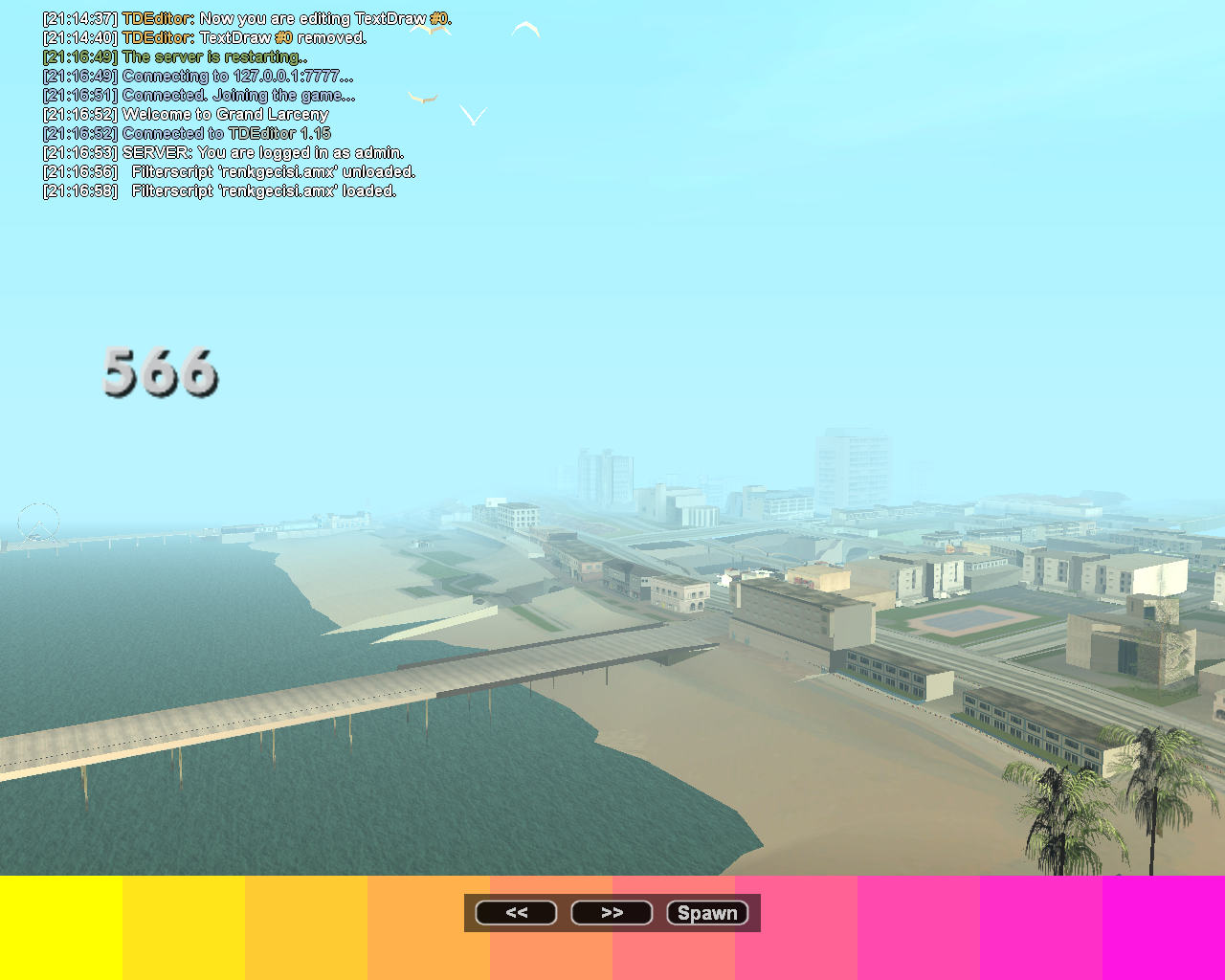
30.09.2017, 18:37
Try more steps so that the transition becomes smooth (about 200 will make each TextDraw about 3 pixels (of 640), which is still a visible transition). It also helps to choose colors that aren't too far away from each other (on the RGB Scale).
But except the colors, position and size of it, it's pretty much the same as in the picture, or am I missing something?
But except the colors, position and size of it, it's pretty much the same as in the picture, or am I missing something?
« Next Oldest | Next Newest »
Users browsing this thread: 1 Guest(s)