16.04.2015, 18:42
Introduction
y_colours is, very simply, defines for 575 different colours. The include has
multiple names for all the colours, and multiple ways of using them too.
You can use the defines anywhere that requires a standard colour:
You can also embed the colours in strings, by using a slightly different name:
Result:

Names
There are several groups of defines in the include, each with different prefixes.
strings, to be used in any SA:MP functions that will take them:
Result:
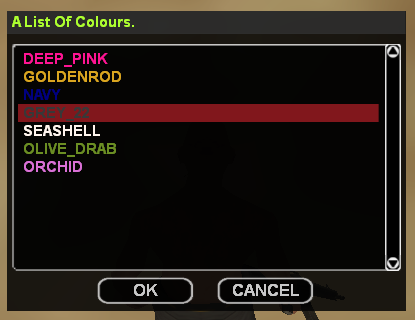
of colours defined for the "X11" system in Linux:
http://en.wikipedia.org/wiki/X11_colors
These are the same as the embeddable colours, but as a standard define with a
fixed alpha of "0xAA" (standard in SA:MP). They are used outside strings when
a colour is required:
same way as the colours with the "X11_" prefix, but have no alpha set at all.
This makes them invisible, but also allows them to have a custom alpha channel
given:
Result:

The bottom left quadrant is there, but invisible. The top right quadrant can't
be seen through at all. The other two are less obvious in their differences,
but they are different and are clearly partially translucent.
Doing "Y_GREEN | 0xAA" is exactly the same as doing "X11_GREEN". The latter has
its alpha hard-coded, the former doesn't.
Result:
y_colours has these colours in it as well. The naming is very similar, only
using "SAMP" instead of "X11":
Result:
Blown up comparison:
Here "X" is used to get the default GameText colour (the brownish-yellow).
There is no "~~" pattern for that exact colour, so it can't be used otherwise.
The full list of these colours are:
this system as "CHARTREUSE" for embedding, "X11_CHARTREUSE" for most other uses,
and "Y_CHARTREUSE" for other uses with a custom alpha channel.
code, so you can either drop them or use an underscore instead. For example
"Yellow Green" becomes either "YELLOW_GREEN" or "YELLOWGREEN" - both are
acceptable. The same applies to those colours with numbers after them - "Royal
Blue 3" is either "ROYAL_BLUE_3" or "ROYALBLUE3".
Note that this doesn't apply to the prefixes. When using "Light Salmon" with a
custom alpha channel, it is either "Y_LIGHTSALMON | 0xFF" or "Y_LIGHT_SALMON | 0xFF",
never "YLIGHTSALMON | 0xFF".
hate the letter "u". Therefore everything in "y_colours" is defined for both
British and American spellings. "Colour" and "color" are both used, as are both
"grey" and "gray". "X11_LIGHT_GRAY" is the same as "X11_LIGHT_GREY" (as well as
"X11_LIGHTGREY" and "X11_LIGHTGRAY").
As a result, the system can be included in one of four different ways:
3.1 best method:
3.1 alternate method:
4.0 best method:
4.0 alternate method:
All do the same things, and the "y_colors" variants simply include the
"y_colours" variants. The 3.1 methods also still work on 4.0.
When using GameText colours normally, the syntax is "~b~~h~" etc. y_colours
adds a "GT" macro to enable this syntax when embedding colours:
Result:
y_text
When using "y_text", all y_colours colours can be directly embedded:
The names here are even more forgiving than the defines listed above. The "X11"
prefix is optional; spaces can be there, not there, or underscores, and the
whole text is case insensitive. These are all equivalent:
List Of Colours
The full list of available colours as an image is here:
https://raw.github.com/Y-Less/YSI/master/y_colours.png
And as a searchable web page is here:
http://y-less.com/YSI/x11.html
The names are given in British spelling WITH spaces, but using the rules above
you can convert these versions to any form you like. For example, the image
contains:
This can be embedded as "MEDIUMAQUAMARINE" or "MEDIUM_AQUAMARINE. Used with a
default alpha value as "X11_MEDIUMAQUAMARINE" or "X11_MEDIUM_AQUAMARINE", and
given an explicit alpha value with "Y_MEDIUMAQUAMARINE | alpha" or
"Y_MEDIUM_AQUAMARINE | alpha".
Similarly "DARK SLATE GREY 3", as it is listed on that grid, is valid as
"X11_DARK_SLATE_GRAY_3", "Y_DARKSLATEGREY3", and multiple other variants.
Adding Colours To y_text
If the provided colours are not enough you can add your own. You may also want
to rename some colours, for example using "ROYAL BLUE" as "HEADING 1" for
separation of content and appearance. The main entry point for this is
"SetColour" (or, as with all the following function "SetColor" if you object to
the "u"):
Very simply just specify the name of the colour and the colour itself. After
calling this function, that colour will be available to "y_text", but not to the
default SA:MP functions like "SendClientMessage";
Using Colours In y_text
The stream parser in y_text can parse a number of different colour
specifications, including:
This uses a "greedy" matching system that is very efficient. This means that doing:
Is equivalent to:
And NOT:
"GREY 100" is a colour, and y_text will find the LONGEST colour it can. Doing:
Will result in "GREY 11" being found - "GREY 110" and "GREY 1100" are not valid
colours, and "GREY 1" (while valid) is not the longest possible colour:
To avoid this, use braces:
You can change y_colours to not ignore spaces with:
In this case then:
Will give the expected:
However, you must still be careful:
"GOLDENROD", aka "GOLDEN ROD" is a colour, but "GOLDEN" isn't. If you use this
string with spaces disabled, then the longest valid colour after the "#" and
before the space is just "GOLD". The result being:
This is probably NOT what is wanted!
y_colours is, very simply, defines for 575 different colours. The include has
multiple names for all the colours, and multiple ways of using them too.
You can use the defines anywhere that requires a standard colour:
pawn Код:
SetPlayerColor(playerid, X11_SNOW);
SendClientMessage(playerid, X11_ROSY_BROWN, "Your colour was set to SNOW");
pawn Код:
SetPlayerColor(playerid, X11_MEDIUM_PURPLE);
SendClientMessage(playerid, X11_CHOCOLATE_2, "Your colour was set to " MEDIUM_PURPLE "MEDIUM PURPLE");

Names
There are several groups of defines in the include, each with different prefixes.
- Embeddable Colours
strings, to be used in any SA:MP functions that will take them:
pawn Код:
ShowPlayerDialog(playerid, 5943, DIALOG_STYLE_LIST,
GREEN_YELLOW "A List Of Colours.",
DEEP_PINK "DEEP_PINK " "\n"\
GOLDENROD "GOLDENROD " "\n"\
NAVY "NAVY " "\n"\
GREY_22 "GREY_22 " "\n"\
SEASHELL "SEASHELL " "\n"\
OLIVE_DRAB "OLIVE_DRAB" "\n"\
ORCHID "ORCHID " "\n",
"OK", "CANCEL");
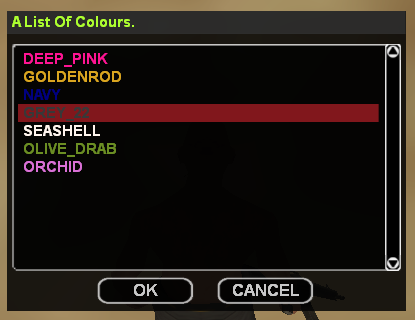
- X11 Colours
of colours defined for the "X11" system in Linux:
http://en.wikipedia.org/wiki/X11_colors
These are the same as the embeddable colours, but as a standard define with a
fixed alpha of "0xAA" (standard in SA:MP). They are used outside strings when
a colour is required:
pawn Код:
TextDrawColor(textDraw, X11_LEMON_CHIFFON_2);
- Y Colours
same way as the colours with the "X11_" prefix, but have no alpha set at all.
This makes them invisible, but also allows them to have a custom alpha channel
given:
pawn Код:
new
z0 = GangZoneCreate(-100.0, -100.0, 0.0, 0.0),
z1 = GangZoneCreate( 0.0, -100.0, 100.0, 0.0),
z2 = GangZoneCreate(-100.0, 0.0, 0.0, 100.0),
z3 = GangZoneCreate( 0.0, 0.0, 100.0, 100.0);
GangZoneShowForPlayer(playerid, z0, Y_MEDIUM_SPRING_GREEN | 0x00); // Invisible.
GangZoneShowForPlayer(playerid, z1, Y_DEEP_SKY_BLUE | 0x55); // Very Translucent.
GangZoneShowForPlayer(playerid, z2, Y_WHEAT_4 | 0xAA); // Less Translucent.
GangZoneShowForPlayer(playerid, z3, Y_THISTLE_3 | 0xFF); // Fully opaque.

The bottom left quadrant is there, but invisible. The top right quadrant can't
be seen through at all. The other two are less obvious in their differences,
but they are different and are clearly partially translucent.
Doing "Y_GREEN | 0xAA" is exactly the same as doing "X11_GREEN". The latter has
its alpha hard-coded, the former doesn't.
- GameText Colours
pawn Код:
GameTextForPlayer(playerid, "Hello~r~ World", 5000, 3);
y_colours has these colours in it as well. The naming is very similar, only
using "SAMP" instead of "X11":
pawn Код:
SendClientMessage(playerid, SAMP_GAMETEXT_X, "Hello" GAMETEXT_R " World");
// OR:
SendClientMessage(playerid, Y_GAMETEXT_X | 0xAA, "Hello" GAMETEXT_R " World");
Blown up comparison:
Here "X" is used to get the default GameText colour (the brownish-yellow).
There is no "~~" pattern for that exact colour, so it can't be used otherwise.
The full list of these colours are:
- SAMP_GAMETEXT_X : ""
- SAMP_GAMETEXT_XH : "~h~"
- SAMP_GAMETEXT_XHH : "~h~~h~"
- SAMP_GAMETEXT_R : "~r~"
- SAMP_GAMETEXT_RH : "~r~~h~"
- SAMP_GAMETEXT_RHH : "~r~~h~~h~"
- SAMP_GAMETEXT_RHHH : "~r~~h~~h~~h~"
- SAMP_GAMETEXT_RHHHH : "~r~~h~~h~~h~~h~"
- SAMP_GAMETEXT_RHHHHH : "~r~~h~~h~~h~~h~~h~"
- SAMP_GAMETEXT_G : "~g~"
- SAMP_GAMETEXT_GH : "~g~~h~"
- SAMP_GAMETEXT_GHH : "~g~~h~~h~"
- SAMP_GAMETEXT_GHHH : "~g~~h~~h~~h~"
- SAMP_GAMETEXT_GHHHH : "~g~~h~~h~~h~~h~"
- SAMP_GAMETEXT_B : "~b~"
- SAMP_GAMETEXT_BH : "~b~~h~"
- SAMP_GAMETEXT_BHH : "~b~~h~~h~"
- SAMP_GAMETEXT_BHHH : "~b~~h~~h~~h~"
- SAMP_GAMETEXT_Y : "~y~"
- SAMP_GAMETEXT_YH : "~y~~h~"
- SAMP_GAMETEXT_YHH : "~y~~h~~h~"
- SAMP_GAMETEXT_P : "~p~"
- SAMP_GAMETEXT_PH : "~p~~h~"
- SAMP_GAMETEXT_PHH : "~p~~h~~h~"
- SAMP_GAMETEXT_W : "~w~"
- SAMP_GAMETEXT_L : "~l~"
- Case
this system as "CHARTREUSE" for embedding, "X11_CHARTREUSE" for most other uses,
and "Y_CHARTREUSE" for other uses with a custom alpha channel.
- Spaces
code, so you can either drop them or use an underscore instead. For example
"Yellow Green" becomes either "YELLOW_GREEN" or "YELLOWGREEN" - both are
acceptable. The same applies to those colours with numbers after them - "Royal
Blue 3" is either "ROYAL_BLUE_3" or "ROYALBLUE3".
Note that this doesn't apply to the prefixes. When using "Light Salmon" with a
custom alpha channel, it is either "Y_LIGHTSALMON | 0xFF" or "Y_LIGHT_SALMON | 0xFF",
never "YLIGHTSALMON | 0xFF".
- Spelling
hate the letter "u". Therefore everything in "y_colours" is defined for both
British and American spellings. "Colour" and "color" are both used, as are both
"grey" and "gray". "X11_LIGHT_GRAY" is the same as "X11_LIGHT_GREY" (as well as
"X11_LIGHTGREY" and "X11_LIGHTGRAY").
As a result, the system can be included in one of four different ways:
3.1 best method:
pawn Код:
#include <YSI\y_colours>
pawn Код:
#include <YSI\y_colors>
pawn Код:
#include <YSI_Server\y_colours>
pawn Код:
#include <YSI_Server\y_colors>
"y_colours" variants. The 3.1 methods also still work on 4.0.
- GameText
- SAMP_GAMETEXT_RH
- SAMP_GAMETEXT_rh
- SAMP_GAME_TEXT_RH
- SAMP_GAME_TEXT_rh
- SAMP_GT_RH
- SAMP_GT_rh
- GAMETEXT_YHH
- GAMETEXT_yhh
- GAME_TEXT_YHH
- GAME_TEXT_yhh
- GT_YHH
- GT_yhh
- Y_GAMETEXT_P | 0xFF
- Y_GAMETEXT_p | 0xFF
- Y_GAME_TEXT_P | 0xFF
- Y_GAME_TEXT_p | 0xFF
- Y_GT_P | 0xFF
- Y_GT_p | 0xFF
When using GameText colours normally, the syntax is "~b~~h~" etc. y_colours
adds a "GT" macro to enable this syntax when embedding colours:
pawn Код:
SendClientMessage(playerid, SAMP_GT_X, "Now for" GT(~g~~h~) " GREEN " GT(~x~) "and" GT(~b~) " BLUE");
y_text
When using "y_text", all y_colours colours can be directly embedded:
Код:
; In a y_text file. Y_TEXT_ENTRY = Hi {ALICEBLUE}there
prefix is optional; spaces can be there, not there, or underscores, and the
whole text is case insensitive. These are all equivalent:
Код:
Hi {light SLATE GREY}there Hi {X11_LIGHT SLATE_GREY}there Hi {X11 LIGHTSLATE GRAY}there Hi {LIGHTSLATEGRaY}there Hi {x11 LIGHT SLatE_gREY}there Hi {X11_LIGht SLATEGrey}there
The full list of available colours as an image is here:
https://raw.github.com/Y-Less/YSI/master/y_colours.png
And as a searchable web page is here:
http://y-less.com/YSI/x11.html
The names are given in British spelling WITH spaces, but using the rules above
you can convert these versions to any form you like. For example, the image
contains:
This can be embedded as "MEDIUMAQUAMARINE" or "MEDIUM_AQUAMARINE. Used with a
default alpha value as "X11_MEDIUMAQUAMARINE" or "X11_MEDIUM_AQUAMARINE", and
given an explicit alpha value with "Y_MEDIUMAQUAMARINE | alpha" or
"Y_MEDIUM_AQUAMARINE | alpha".
Similarly "DARK SLATE GREY 3", as it is listed on that grid, is valid as
"X11_DARK_SLATE_GRAY_3", "Y_DARKSLATEGREY3", and multiple other variants.
Adding Colours To y_text
If the provided colours are not enough you can add your own. You may also want
to rename some colours, for example using "ROYAL BLUE" as "HEADING 1" for
separation of content and appearance. The main entry point for this is
"SetColour" (or, as with all the following function "SetColor" if you object to
the "u"):
pawn Код:
native SetColour(const name[], color); // Or "SetColor".
calling this function, that colour will be available to "y_text", but not to the
default SA:MP functions like "SendClientMessage";
pawn Код:
SetColour("ALMOST RED", 0xF01010AA);
Код:
; In a y_text file. ANOTHER_TEXT_ITEM = {ALMOST RED}This is in our new colour.
The stream parser in y_text can parse a number of different colour
specifications, including:
Код:
Hello#AZURE There Hello{AZURE} There Hello~w~ There
Код:
I can count to#GREY 100!
Код:
I can count to{FFFFFF}!
Код:
I can count to{BEBEBE} 100!
Код:
I can count to#GREY 1100!
colours, and "GREY 1" (while valid) is not the longest possible colour:
Код:
I can count to{1C1C1C}00!
Код:
I can count to{GRAY} 100!
pawn Код:
SetColoursCanHaveSpaces(false);
Код:
I can count to#GRAY 100!
Код:
I can count to{BEBEBE} 100!
Код:
I can count to#GOLDEN ROD 100!
string with spaces disabled, then the longest valid colour after the "#" and
before the space is just "GOLD". The result being:
Код:
I can count to{FFD700}EN ROD 100!