12.06.2018, 12:01
INTRODUCTION
In this tutorial I will teach you how to create a simple email confirmation system. It will send an email to the player with a code, and he needs to enter that code in the sever in order to play. It is really simple.
You will need first to download SAMPMailJS include HERE.
After you setup the include correctly, let's jump to the tutorial. Note that there are several ways of doing this. The way I'm doing this for the tutorial will be:
- Player registers and enters a valid email
- The server will send an email to the player with a code
- The player can play the first time without confirming the email (you can create a command to do this aswell)
- The second time the player joins the server, it will ask for the code
- If he enters the code correctly, it will confirm the email and let him play, otherwise it will kick him, or anything you want. You can choose not to kick him, but instead block some of the server functionalities for unconfirmed players. It's up to you really.
TUTORIAL
Create 3 new variables on your player enum (normally): (in this case the code will be an integer, you can use a string if you want)
At the registration time, you will generate a new confirmation code, like this:
This code will generate a confirmation code between 1000 and 9999. You can choose to be a bigger or smaller number, it is up to you.
Now let's create the script that will send the email. I will assume you already have SAMPMailJS set up with the last version. That already comes with a confirmation code email template, that we will use here. You can create your own or edit the existing one.
Create a function that sends the email, and call it after you generate the confirmation code:
Now the code to show the dialog to enter the confirmation code. It will appear when the player logins to the server, or if you want create a command to show it:
And it's done. It is that simple.
Email recieved:
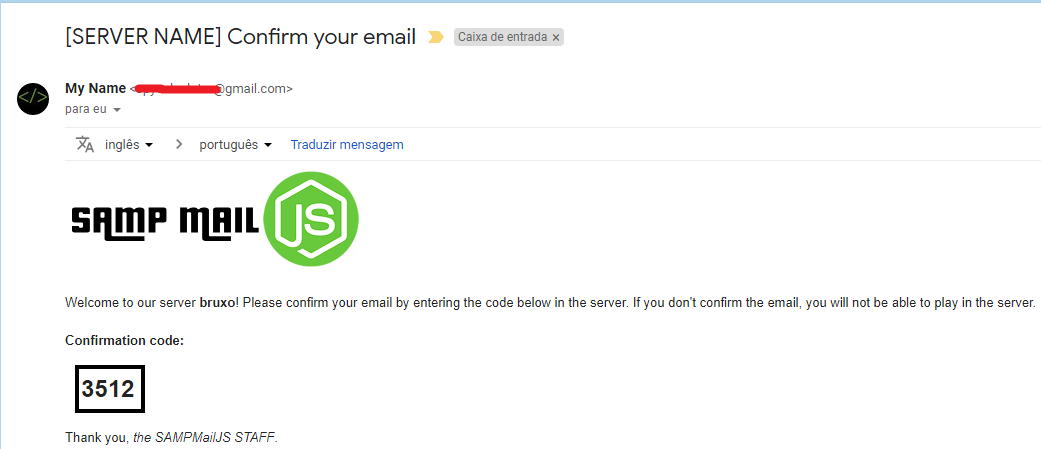
I didn't compiled the code, it may have some syntax errors. If it does, tell me and I will fix it.
In this tutorial I will teach you how to create a simple email confirmation system. It will send an email to the player with a code, and he needs to enter that code in the sever in order to play. It is really simple.
You will need first to download SAMPMailJS include HERE.
After you setup the include correctly, let's jump to the tutorial. Note that there are several ways of doing this. The way I'm doing this for the tutorial will be:
- Player registers and enters a valid email
- The server will send an email to the player with a code
- The player can play the first time without confirming the email (you can create a command to do this aswell)
- The second time the player joins the server, it will ask for the code
- If he enters the code correctly, it will confirm the email and let him play, otherwise it will kick him, or anything you want. You can choose not to kick him, but instead block some of the server functionalities for unconfirmed players. It's up to you really.
TUTORIAL
Create 3 new variables on your player enum (normally): (in this case the code will be an integer, you can use a string if you want)
Code:
Email[32], ConfCode, Confirmed
Code:
stock RandomEx(min, max) // ****** | returns a int between min and max { new rand = random(max - min) + min; return rand; } // Put this in your registration system, when the player enters his email Player[playerid][ConfCode] = RandomEx(1000, 9999); // code will be between 1000 and 9999 Player[playerid][Confirmed] = 0; // player is not confirmed
Now let's create the script that will send the email. I will assume you already have SAMPMailJS set up with the last version. That already comes with a confirmation code email template, that we will use here. You can create your own or edit the existing one.
Create a function that sends the email, and call it after you generate the confirmation code:
Code:
forward SendConfirmationEmail(playerid); public SendConfirmationEmail(playerid) { new text[64], pName[MAX_PLAYER_NAME]; GetPlayerName(playerid, pName, sizeof pName); format(text, sizeof text, "PNAME:%s#CONFCODE:%d", pName, Player[playerid][ConfCode]); // formats the string with the code standard that SAMPMailJS accepts. tag1:value1#tag2:value2 SendEmail("My Server Name", Player[playerid][Email], "[SERVER NAME] Confirm your email", text, true, "confcode.html"); return 1; }
Code:
#define DIALOG_CONFIRM 500 // define a dialog ID // After the player logins if(Player[playerid][Confirmed] == 0) { return ShowPlayerDialog(playerid, DIALOG_CONFIRM, DIALOG_STYLE_INPUT, "CONFIRM YOUR EMAIL", "Email not confirmed. Enter the confirmation code sent to your email.", "Confirm", "Cancel"); } // OnDialogResponse(...) case DIALOG_CONFIRM: { if(!response) return Kick(playerid); if(Player[playerid][ConfCode] == strval(inputtext)) { // if the code and the inputtext are the same SendClientMessage(playerid, -1, "You confirmed your email!"); Player[playerid][Confirmed] = 1; // player confirmed } else { // otherwise Kick(playerid); // kicks the player } }
Email recieved:
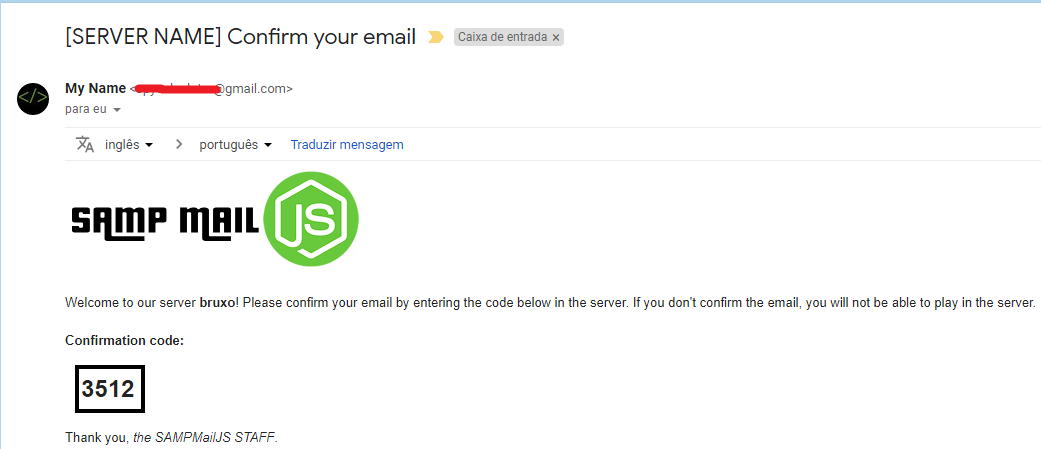
I didn't compiled the code, it may have some syntax errors. If it does, tell me and I will fix it.