22.12.2015, 14:42
(
Последний раз редактировалось iSteve; 13.12.2016 в 05:14.
)
Stacks
Hello there,In this tutorial you are going to learn what the stacks really are.We are going to utilize the concept of arrays to help us out with this,however, a little knowledge in the concept of arrays is highly appritiated.
I recommend the players to read https://sampforum.blast.hk/showthread.php?tid=318212 before starting off with stacks and queues
First off lets start by what an array actually is..
Array
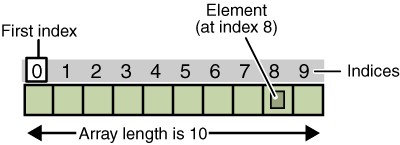
Initializing Arrays
Arrays in pawn can be initialized in the following way
Код:
new a[10];
Accessing Array Elements
An element is accessed by indexing the array name. This is done by placing the index of the element within square brackets after the name of the array. Eg
Код:
a[1]=50; a[2]=38;
now you can use a[2] anywhere where you use 38,just like a variable.
Not going to further details about arrays,lets start with stacks and queues.
Stacks

The stacks consists of 2 primary operations.
- The push function:Will add the element on the top of the stack of arrays.
- The pop function:Will remove the element from the top of the stack of arrays
A variable is required to know where the push and pop functions are going to work
Код:
#declare MAX 5 //We are going to say that 5 is the max ammount of variables that we are stacking. new stack[MAX]; new top=-1;//The variable we are going to use to locate where our point of insertion or deletion is
When we push the element into an array,we must see if the array has maximum number of element,this is where our variable top comes into play, if top is equal to MAX-1(since array starts from 0) we will say that the is at its full and this is thus our overflow condition.
We will return 1 if the push function works and -9999 if it fails.
Код:
stock push(new item,new a[])//Parameters are the element to be insert & array { if(top==MAX-1)//THE overflow condition. { printf("[Error]:Stack Overflow!\n"); return -9999; } top=top+1; a[top]= item; return 1; }
alternatively the code can be substituted with a single line of code
Quote:
top=top+1; a[top]= item; |
Quote:
a[++top]=item; |
Pop function
When we pop the element from an array,we must see if the array is empty,this is where our variable top comes into play, if top is equal to -1(since array starts from 0) we will say that the is at its empty and this is thus our underflow condition.
We will return the popped element if it did happen succesfully and -9999 if the operation failed.
Код:
stock pop(new a[])//Parameters is the array { new item; if(top==-1) { printf("[ERROR]:Stack underflow!"); return -9999; } item=a[top] top=top-1; return item; }
Quote:
item=a[top] top=top-1; |
Quote:
item=a[top--]; |