15.08.2016, 20:49
Crash detect error:
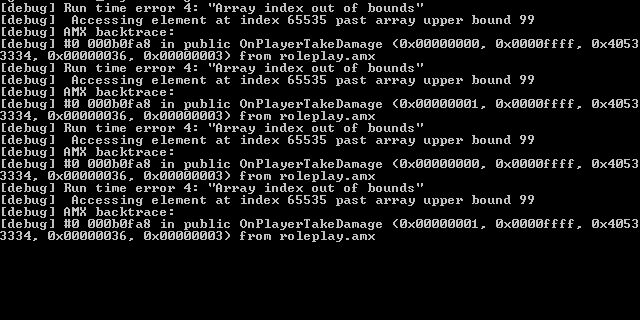
I've got no clue on what's wrong.
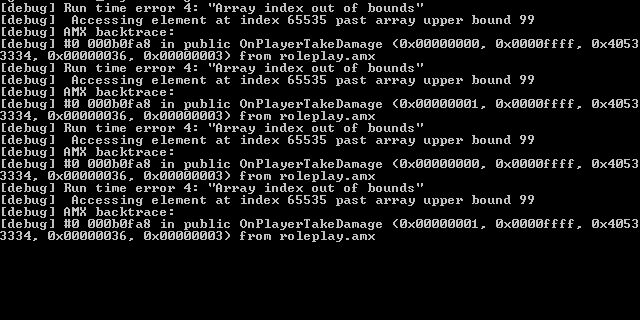
Код:
public OnPlayerTakeDamage(playerid, issuerid, Float:amount, weaponid, bodypart) { new Float: health; new Float: armour; GetPlayerHealth(playerid, health); GetPlayerArmour(playerid, armour); if(weaponid == 24) { switch(armour) { case 0: { switch(bodypart) { case 3: SetPlayerHealth(playerid, health - 25); // Torso. case 4: SetPlayerHealth(playerid, health - 20); // Groin. case 5: SetPlayerHealth(playerid, health - 10); // Left Arm. case 6: SetPlayerHealth(playerid, health - 10); // Right Arm. case 7: SetPlayerHealth(playerid, health - 10); // Left Leg. case 8: SetPlayerHealth(playerid, health - 10); // Right Leg. case 9: SetPlayerHealth(playerid, health - 50); // Head. } } default: { switch(bodypart) { case 3: SetPlayerArmour(playerid, armour - 13); // Torso. case 4: SetPlayerArmour(playerid, armour - 10); // Groin. case 5: SetPlayerArmour(playerid, armour - 5); // Left Arm. case 6: SetPlayerArmour(playerid, armour - 5); // Right Arm. case 7: SetPlayerArmour(playerid, armour - 5); // Left Leg. case 8: SetPlayerArmour(playerid, armour - 5); // Right Leg. case 9: SetPlayerArmour(playerid, armour - 25); // Head. } } } } else if(weaponid == 25) { switch(armour) // Creates a switch that switches through the armour float, and checks the value. { case 0: // If the value is 0, the codes underneath will activate. { switch(bodypart) { case 3: SetPlayerHealth(playerid, health - 40); // Torso. case 4: SetPlayerHealth(playerid, health - 20); // Groin. case 5: SetPlayerHealth(playerid, health - 15); // Left Arm. case 6: SetPlayerHealth(playerid, health - 15); // Right Arm. case 7: SetPlayerHealth(playerid, health - 15); // Left Leg. case 8: SetPlayerHealth(playerid, health - 15); // Right Leg. case 9: SetPlayerHealth(playerid, health - 100); // Head. } } default: // Otherwise the codes underneath will activate. { switch(bodypart) { case 3: SetPlayerArmour(playerid, armour - 20); // Torso. case 4: SetPlayerArmour(playerid, armour - 10); // Groin. case 5: SetPlayerArmour(playerid, armour - 7); // Left Arm. case 6: SetPlayerArmour(playerid, armour - 7); // Right Arm. case 7: SetPlayerArmour(playerid, armour - 7); // Left Leg. case 8: SetPlayerArmour(playerid, armour - 7); // Right Leg. case 9: SetPlayerArmour(playerid, armour - 50); // Head. } } } } else if(weaponid == 30) { switch(armour) // Creates a switch that switches through the armour float, and checks the value. { case 0: // If the value is 0, the codes underneath will activate. { switch(bodypart) { case 3: SetPlayerHealth(playerid, health - 35); // Torso. case 4: SetPlayerHealth(playerid, health - 35); // Groin. case 5: SetPlayerHealth(playerid, health - 20); // Left Arm. case 6: SetPlayerHealth(playerid, health - 20); // Right Arm. case 7: SetPlayerHealth(playerid, health - 14); // Left Leg. case 8: SetPlayerHealth(playerid, health - 14); // Right Leg. case 9: SetPlayerHealth(playerid, health - 50); // Head. } } default: // Otherwise the codes underneath will activate. { switch(bodypart) { case 3: SetPlayerArmour(playerid, armour - 15); // Torso. case 4: SetPlayerArmour(playerid, armour - 15); // Groin. case 5: SetPlayerArmour(playerid, armour - 10); // Left Arm. case 6: SetPlayerArmour(playerid, armour - 10); // Right Arm. case 7: SetPlayerArmour(playerid, armour - 7); // Left Leg. case 8: SetPlayerArmour(playerid, armour - 7); // Right Leg. case 9: SetPlayerArmour(playerid, armour - 50); // Head. } } } } else if(weaponid == 31) { switch(armour) // Creates a switch that switches through the armour float, and checks the value. { case 0: // If the value is 0, the codes underneath will activate. { switch(bodypart) { case 3: SetPlayerHealth(playerid, health - 20); // Torso. case 4: SetPlayerHealth(playerid, health - 20); // Groin. case 5: SetPlayerHealth(playerid, health - 10); // Left Arm. case 6: SetPlayerHealth(playerid, health - 10); // Right Arm. case 7: SetPlayerHealth(playerid, health - 7); // Left Leg. case 8: SetPlayerHealth(playerid, health - 7); // Right Leg. case 9: SetPlayerHealth(playerid, health - 100); // Head. } } default: // Otherwise the codes underneath will activate. { switch(bodypart) { case 3: SetPlayerArmour(playerid, armour - 10); // Torso. case 4: SetPlayerArmour(playerid, armour - 10); // Groin. case 5: SetPlayerArmour(playerid, armour - 5); // Left Arm. case 6: SetPlayerArmour(playerid, armour - 4); // Right Arm. case 7: SetPlayerArmour(playerid, armour - 4); // Left Leg. case 8: SetPlayerArmour(playerid, armour - 4); // Right Leg. case 9: SetPlayerArmour(playerid, armour - 50); // Head. } } } } else if(weaponid == 34) { switch(armour) // Creates a switch that switches through the armour float, and checks the value. { case 0: // If the value is 0, the codes underneath will activate. { switch(bodypart) { case 3: SetPlayerHealth(playerid, health - 50); // Torso. case 4: SetPlayerHealth(playerid, health - 25); // Groin. case 5: SetPlayerHealth(playerid, health - 10); // Left Arm. case 6: SetPlayerHealth(playerid, health - 10); // Right Arm. case 7: SetPlayerHealth(playerid, health - 10); // Left Leg. case 8: SetPlayerHealth(playerid, health - 10); // Right Leg. case 9: SetPlayerHealth(playerid, health - 100); // Head. } } default: // Otherwise the codes underneath will activate. { switch(bodypart) { case 3: SetPlayerArmour(playerid, armour - 25); // Torso. case 4: SetPlayerArmour(playerid, armour - 15); // Groin. case 5: SetPlayerArmour(playerid, armour - 5); // Left Arm. case 6: SetPlayerArmour(playerid, armour - 5); // Right Arm. case 7: SetPlayerArmour(playerid, armour - 5); // Left Leg. case 8: SetPlayerArmour(playerid, armour - 5); // Right Leg. case 9: SetPlayerArmour(playerid, armour - 50); // Head. } } } } if(actmarker[issuerid] >= 1) { TextDrawShowForPlayer(issuerid, HitMarker); timarker = SetTimerEx("HTD", 250, true, "%d", issuerid); } if (PlayerData[playerid][pFirstAid]) { SendClientMessage(playerid, COLOR_LIGHTRED, "[WARNING]:{FFFFFF} Your first aid kit is no longer in effect as you took damage."); PlayerData[playerid][pFirstAid] = 0; KillTimer(PlayerData[playerid][pAidTimer]); } return 1; }